6.2.7 - SUI Sliders
A slider in SUI is defined as SSlider. To create a slider we add it to a panel or container as usual.
For it to work we need to use the .Notify method passing a method (action) which takes a float parameter like so:
public static void Create()
{
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SSlider.Text("Slider").Notify(PrintSlider); // passing a method which takes a float parameter
panel.Add(container);
}
private static void PrintSlider(float number) // receiving the slider value
{
RLog.Msg(number); // printing the slider value
}
Now, whenever we change the value of the slider, the PrintSlider
method will be called.
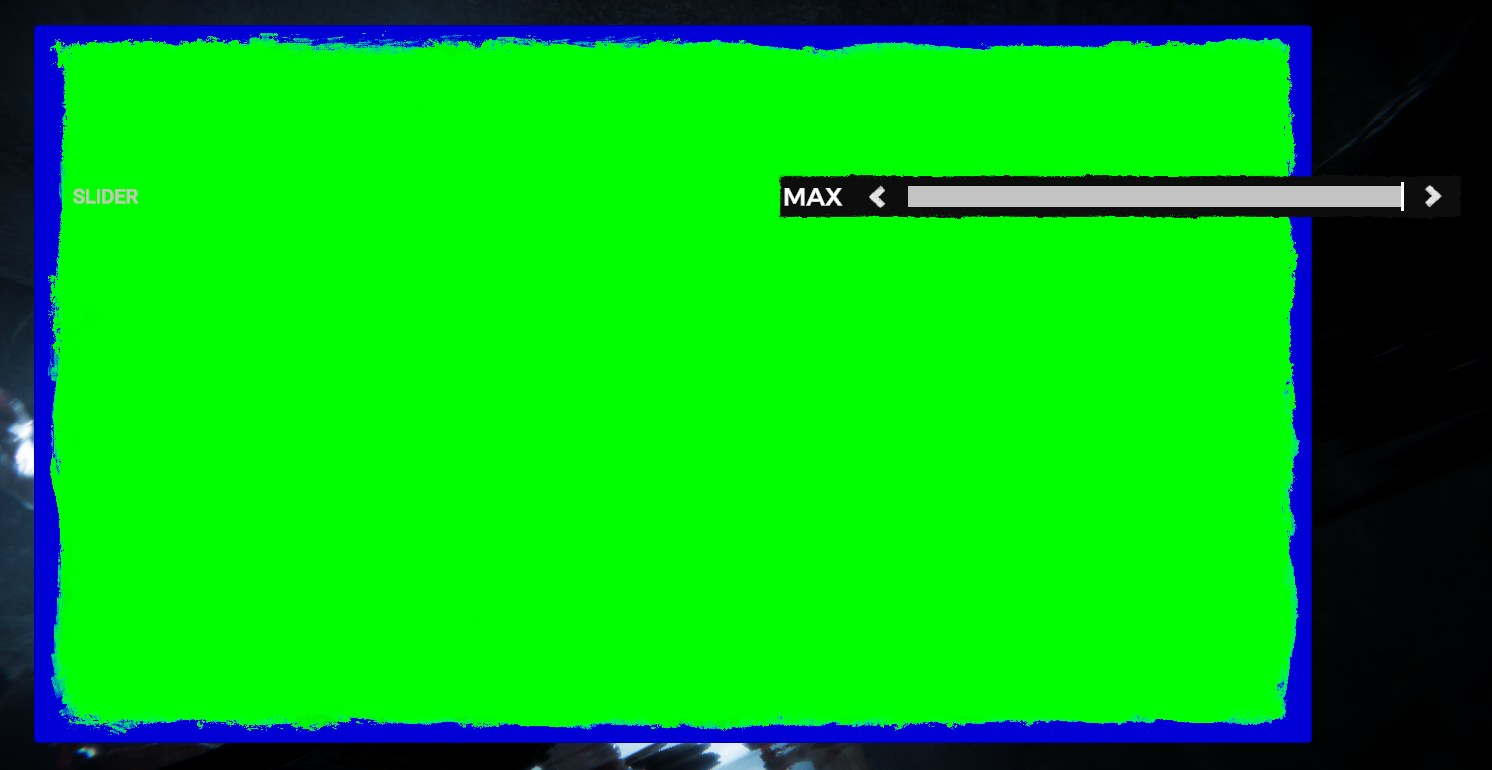
Setting a range
To set a range of min and max value of the slider we can use the .Range method like so:
SSlider.Text("Slider").Range(0, 10).Notify(PrintSlider); // slider with a range of 0 to 10
Setting decimal precision
To change how many numbers are after comma we can use the .Format method like so:
SSlider.Text("Slider").Format("0.0").Notify(PrintSlider); // slider with just one number after comma using the "Format" method
Setting slider step value
To change how much the value of the slider should change when dragging it we can use the .Step method like so:
SSlider.Text("Slider").Step(0.5f).Notify(PrintSlider); // number will increase by 0.5 each step
We can also make it of type integer using the .IntStep method, so it will ignore decimals:
SSlider.Text("Slider").IntStep().Notify(PrintSlider); // skipping decimal values